我们来看一段代码和执行结果:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| package com.douya.Test; * Created by douya on 17-4-25. */ public class OrderTest { public static void main(String[] args) { new B(); } public static class A { static { System.out.println("static A"); } { System.out.println("codeBlock A"); } public A() { System.out.println("constructor A"); } } public static class B extends A { static { System.out.println("static B"); } { System.out.println("codeBlock B"); } public B() { System.out.println("constructor B"); } } }
|
执行结果:
static A
static B
codeBlock A
constructor A
codeBlock B
constructor B
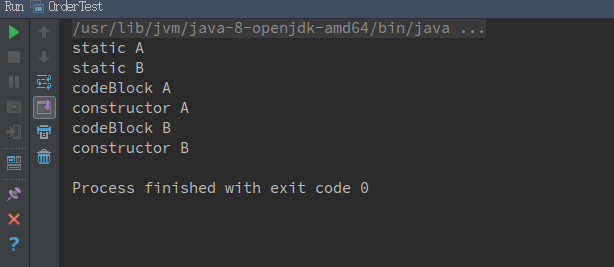
结论:
两大原则:
- 父类先行
- 静态方法先行
故而执行顺序为:父类静态方法->子类静态方法->父类代码块->父类构造方法->子类代码块->子类构造方法
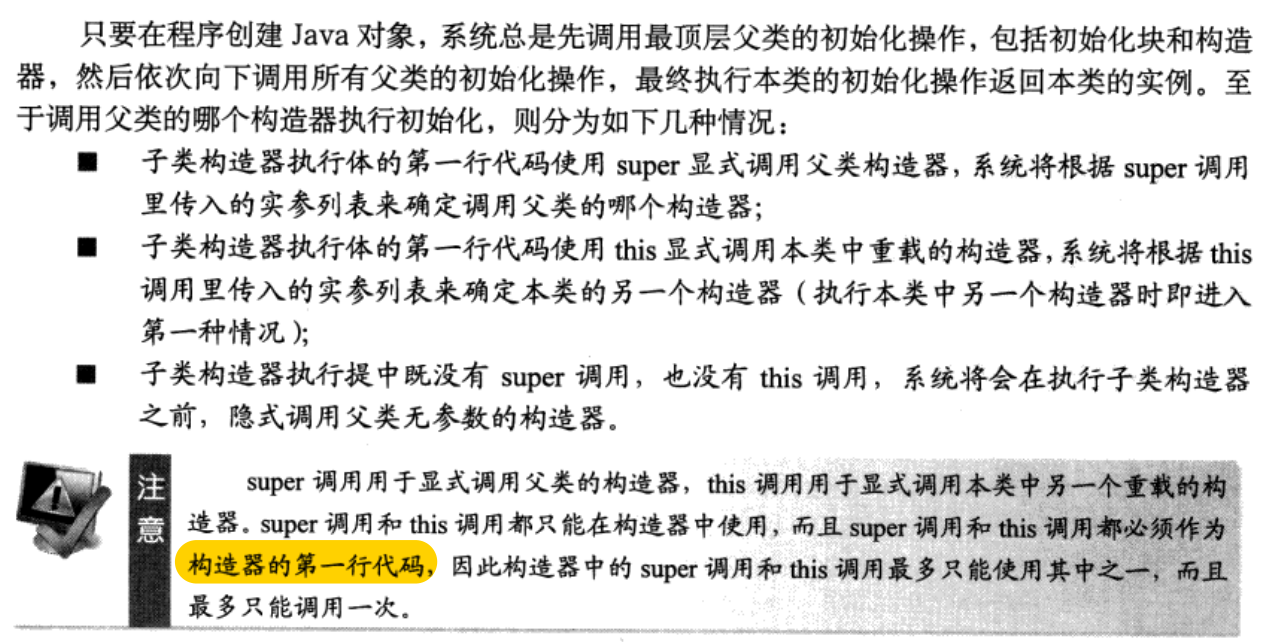
补充
看如下代码和运行结果:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| package com.douya.day9; * Created by douya on 17-5-3. */ class Base { private int i = 2; public Base() { System.out.println(this); this.display(); } public void display() { System.out.println(i); } } class Derived extends Base { private int i = 22; public Derived() { i = 222; } @Override public void display() { System.out.println(i); } } public class WhoIsThis { public static void main(String[] args) { new Derived(); } }
|
com.douya.day9.Derived@3fee733d
0
如果你熟悉子父类的创建和调用就好办了,在new 子类时,this指向的是子类,调用方法时,子类方法覆盖父类同名方法,父类与子类如果有同名属性,则调用的属性要看调用的主体是谁,是父类那就是父类的属性,是子类就是子类的属性。这里display()调用子类display,i是子类的i,子类i在 此时还未被赋值,故其值为0.
练习题
相信你一定懂了,那么我们一起来巩固一下吧~
下面程序的运行结果是什么呢?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
| package com.douya.day9; import com.sun.org.apache.bcel.internal.generic.DDIV; * Created by douya on 17-5-3. */ class BBase { int count = 2; public void display() { System.out.println(this.count); } } class DDerived extends BBase { int count = 20; @Override public void display() { System.out.println(this.count); } } public class BaseAndDerived { public static void main(String[] args) { BBase bBase = new BBase(); System.out.println(bBase.count); bBase.display(); DDerived dDerived = new DDerived(); System.out.println(dDerived.count); dDerived.display(); BBase bd = new DDerived(); System.out.println(bd.count); bd.display(); BBase b2d = dDerived; System.out.println(b2d.count); } }
|
答案:
2
2
20
20
2
20
Tips:
内存中并不存在所有的父类,只是子类保留了父类的所定义的所有实例变量,拥有父类的数据结构